Deposit report component
The deposit report component displays deposits for a given Merchant. Each deposit can be opened to display additional details and actions associated with the deposit, such as the deposit amount and the payments that were included in the deposit.
The deposit report component can be utilized for a variety of reports based on your use case. The report must be shown for a single merchant. Additionally, the report can be filtered by various fields, such as status or date. Clicking on any deposit generates a deposit detail view.
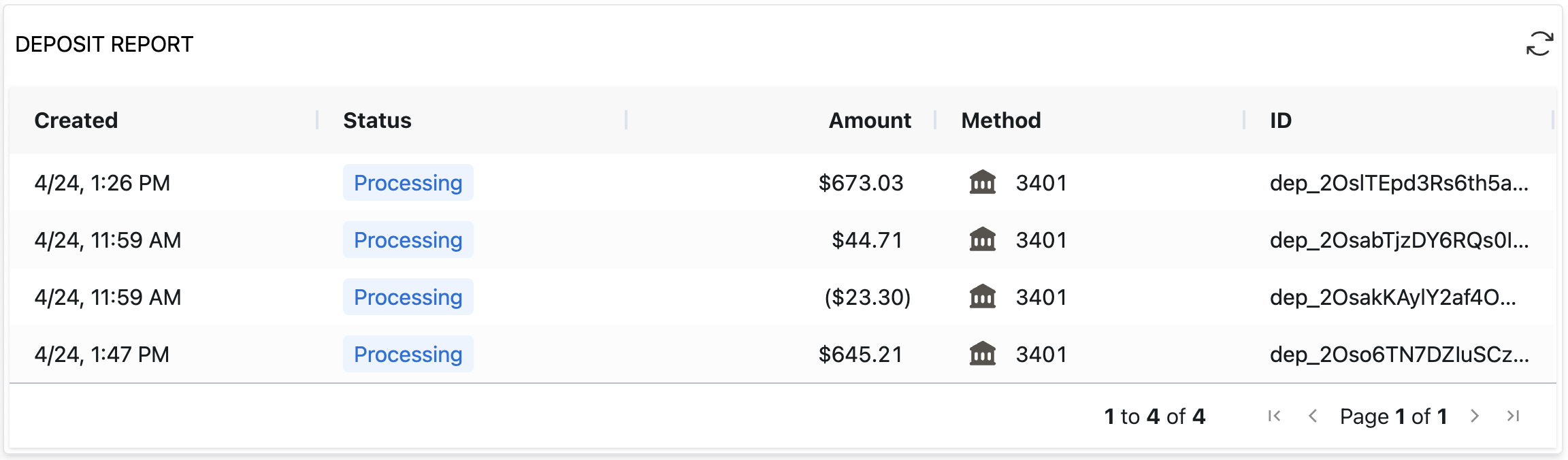
How to embed a deposit report
Session authentication
Create a session to grant the deposit report component permissions to view deposits and the payments that make up a deposit. The session should include at least the following to view deposits and their details:
curl --location 'https://api.rainforestpay.com/v1/sessions' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {{api_key}}' \
--data '{
"ttl": 86400,
"statements": [
{
"permissions": ["group#deposit_report_component"]
}
]
}'
Update permissions are necessary if the user should be able to perform actions such as refunding a payin. The session should include at least the following:
curl --location 'https://api.rainforestpay.com/v1/sessions' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {{api_key}}' \
--data '{
"ttl": 86400,
"statements": [
{
"permissions": [
"group#deposit_report_component",
"group#deposit_report_component.create_refund"
]
}
]
}'
JavaScript bundle
Include the merchant JavaScript bundle in your frontend to access the deposit report component.
<script type="module" src="https://static.rainforestpay.com/sandbox.merchant.js"></script>
Deposit report component
Add the Rainforest deposit report component with custom configuration designed in the component studio.
<rainforest-deposit-report
session-key="REPLACE_ME"
data-filters='{"merchant_id":"sbx_mid_example"}'
></rainforest-deposit-report>
Merchant specification
In order to render the deposit report, a Merchant ID must be provided in the data-filters
attribute. This will filter the report to show deposits for a specific merchant.
<rainforest-deposit-report
session-key="REPLACE_ME"
data-filters='{"merchant_id":"sbx_mid_example"}'
></rainforest-deposit-report>
Column configuration
The deposit report will load with a set of default columns. Simply omit the columns
prop to use the following default columns.
[
{"name":"Created","type":"builtin","value":"created"},
{"name":"Status","type":"builtin","value":"status"},
{"name":"Amount","type":"builtin","value":"amount"},
{"name":"Method","type":"builtin","value":"method"},
{"name":"ID","type":"builtin","value":"id"}
]
Custom configuration
Columns can be customized to fit the needs of your platform. To override the default columns for the deposit report, pass in the column specifications via a JSON payload in the columns
prop.
<rainforest-deposit-report
columns='[{"name":"My Custom Column Name","type":"builtin","value":"id"}]'
></rainforest-deposit-report>
A column specification needs a name
, type
, and value
.
name
- The display header name for the column.type
- Set tobuiltin
value
- The key corresponding to a value returned from the list deposits endpoint.
Valid column values
Type | Value | Description |
---|---|---|
builtin | created | The datetime the deposit was created at. Corresponds to the created_at field. |
builtin | amount | The amount of the deposit. Corresponds to the amount field. |
builtin | method | The payment method for the deposit. Corresponds to the method_type field and the last 4 digits of the account. |
builtin | status | The status of the deposit. Corresponds to the status field. |
builtin | id | The unique identifier for the deposit. Corresponds to the deposit_id field. |
Activity column configuration
The deposit activity report in the deposit details will load with a set of default columns. Simply omit the activity-columns
prop to use the following default columns.
[
{"name":"Created","type":"builtin","value":"created_at"},
{"name":"Type","type":"builtin","value":"type"},
{"name":"ID","type":"builtin","value":"id"},
{"name":"Amount","type":"builtin","value":"gross_amount"},
{"name":"Fees","type":"builtin","value":"billing_fees_amount"},
{"name":"Net","type":"builtin","value":"net_amount"}
]
Custom configuration
Columns can be customized using the same syntax as the deposit report columns. To override the default columns for the deposit activity report, pass in the column specifications via a JSON payload in the activity-columns
prop.
<rainforest-deposit-report
activity-columns='[{"name":"My Custom Column Name","type":"builtin","value":"id"}]'
></rainforest-deposit-report>
A column specification needs a name
, type
, and value
.
name
- The display header name for the column.type
- Set tobuiltin
ormetadata
value
- The key corresponding to a value returned from the deposit activity endpoint.
Valid column values
Type | Value | Description |
---|---|---|
builtin | created_at | The datetime the activity was created at. Corresponds to the created_at field. |
builtin | type | The type of activity. Corresponds to the type field. |
builtin | id | The unique identifier for the activity. Corresponds to the id field. |
builtin | gross_amount | The gross amount of the activity. Corresponds to the gross_amount field. |
builtin | billing_fees_amount | The amount of the billing fees for the activity. Corresponds to the billing_fees_amount field. This column will be omitted if the merchant is configured for gross billing. |
builtin | split_remainder_amount | The sum of the amount splits for the activity. Corresponds to the split_remainder_amount field. |
builtin | net_amount | The net amount due to the merchant for the activity, less any fees and amount splits. Corresponds to the net_amount field. This column will be omitted if the merchant is configured for gross billing. |
builtin | merchant_id | The unique identifier for the merchant associated to the activity. Corresponds to the merchant_id field. |
builtin | merchant_name | The merchant's name associated to the activity. Corresponds to the merchant.name field. |
builtin | payment_method | The payment method for the activity. Corresponds to the payment_method.method_type field and last 4 digits of the account. |
metadata | JSONPath to metadata key | The metadata for the activity. Corresponds to the metadata field. |
Metadata column
A column on the deposit activity report can be configured to display a custom metadata stored on the activity. The value
for the type metadata
should be valid JSONPath to a corresponding metadata key.
For example, payins with the following metadata:
{
"account": {
"id": "123",
"name": "Jane Doe",
"email": "[email protected]
}
}
could have the following column specification to display the metadata account id
{
"name": "Account ID",
"type": "metadata",
"value": "$.account.id"
}
Data filters
The payment report can be pre-loaded with a defined set of filters, including sort by and sort order. Data filters are defined by a JSON payload of key-value pairs passed into the data-filters
prop.
A valid key
includes any of the query params from the list deposits endpoint. To pass multiple values for a given key, the value can be defined as an array of values.
{
"merchant_id": "mid_yourmerchant",
"status": ["PROCESSING", "FAILED"],
"sort_by": "created_at",
"sort_order": "desc"
}
Deposit report component studio
Check out the deposit report component studio to configure and design your deposit report to fit your use case. The custom columns, data filters, and CSS variables are passed to the component through HTML attributes.
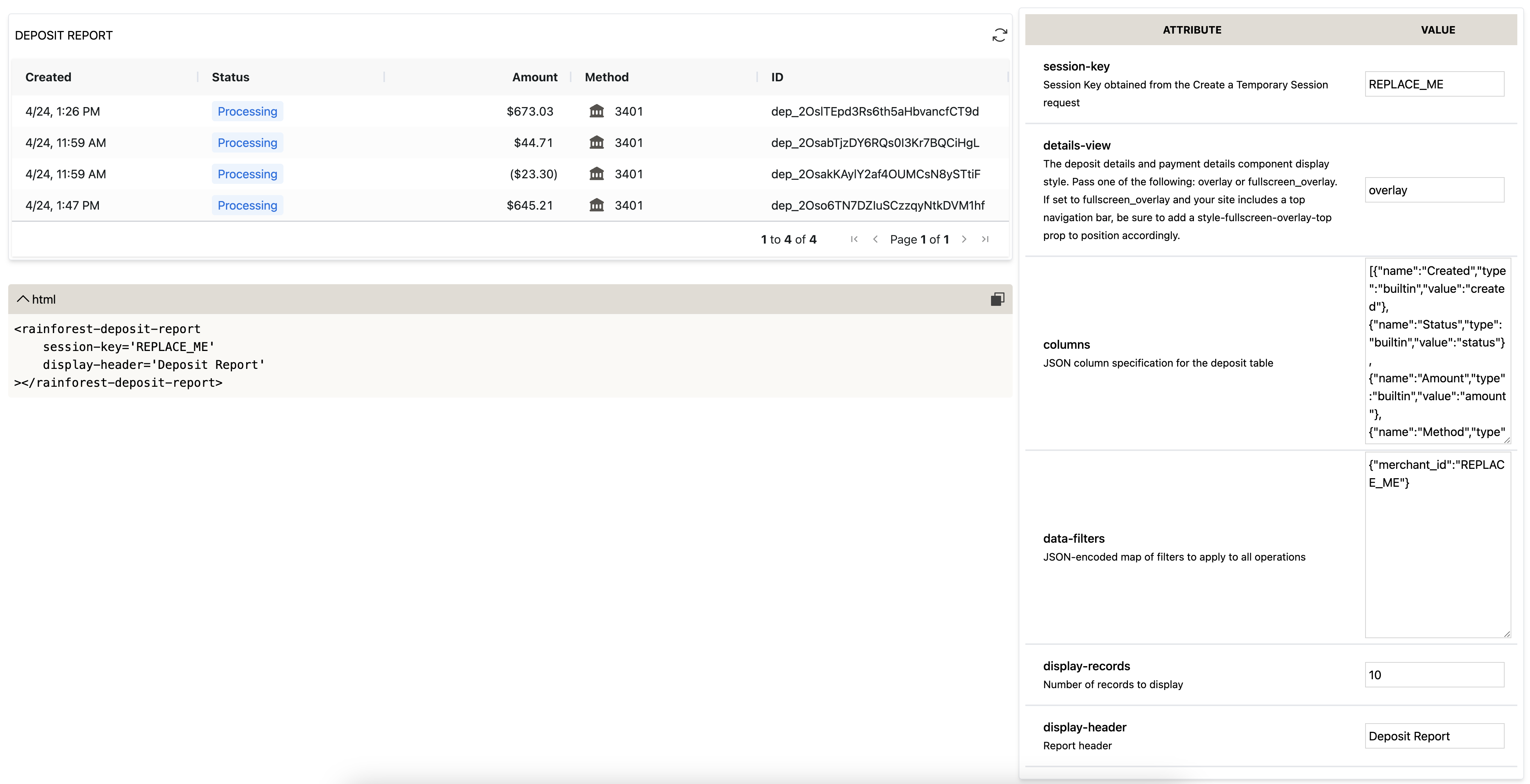
Default attributes
Simply omit the props to use the default attributes. Here is a list of the default attributes:
<rainforest-deposit-report
columns='[{"name":"ID","type":"builtin","value":"id"},{"name":"Status","type":"builtin","value":"state"},{"name":"Amount","type":"builtin","value":"amount"},{"name":"Bank Name","type":"builtin","value":"bank_name"},{"name":"Account","type":"builtin","value":"bank_account"},{"name":"Date","type":"builtin","value":"date"},{"name":"Updated","type":"builtin","value":"date"}]'
data-filters='{"merchant_id": "mid_yourmerchant"}'
show-search
display-records='10'
display-header='Report'
style-font-size='1rem'
style-border-color='#e5e7eb'
style-border-radius='0.5rem'
style-button-color='#303030'
style-button-border-radius='0.5rem'
style-icon-color='#707070'
style-table-color='#808080'
style-table-font-size='0.8rem'
></rainforest-payment-report>
UI Customization
It's possible to provide customized UI to the Deposit Report through our exposed slots. You can read more about web component slots here.
Available Slots
Name | Description |
---|---|
details-loading-indicator | Overrides the default UI shown while loading deposit details |
usage:
<rainforest-deposit-report [...props]>
<div slot="details-loading-indicator">
(your custom UI markup)
</div>
</rainforest-deposit-repot>
Updated 3 months ago