Payment report component
Embed the Rainforest payment report component
The payment report component displays payins and refunds (collectively, payments). Each payment can be opened to display additional details and actions associated to the payment, such as refunding a payin.
The payment report component can be utilized for a variety of reports based on your use case. The report can be filtered by various fields, such as status, date, payment type, or metadata (i.e. customer name, product ID, sales channel, etc.). Clicking on any payment generates a payment detail view.
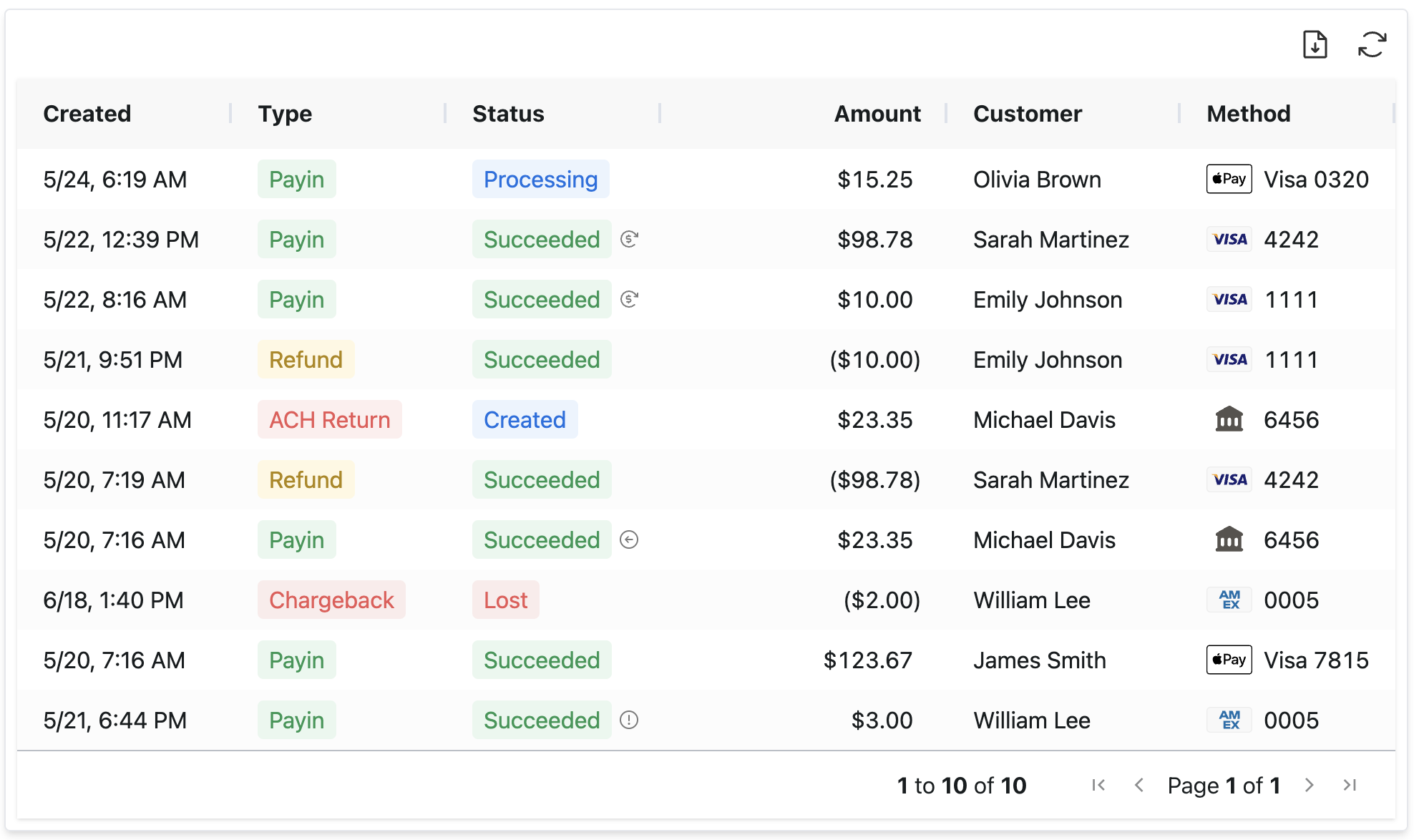
How to embed a payment report
Session authentication
Create a session to grant the payment report component permissions to view and action payments. The session should include at least the following permissions to view payments:
curl --location 'https://api.rainforestpay.com/v1/sessions' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {{api_key}}' \
--data '{
"ttl": 86400,
"statements": [
{
"permissions": ["group#payment_report_component"]
}
]
}'
Session constraints
If the component permissions are constrained to a specific resource, data filters will need to be applied to give the payment report component the same level of permissions.
Commonly, the payment report is pre-loaded to show payments for a single merchant. The session should be constrained to a specific merchant to ensure this session only has permissions to view the payments associated to the merchant:
curl --location 'https://api.rainforestpay.com/v1/sessions' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {{api_key}}' \
--data '{
"ttl": 86400,
"statements": [
{
"permissions": [
"group#payment_report_component",
],
"constraints": {
"merchant: {
"merchant_id": "REPLACE_ME"
}
}
}
]
}'
Refund permissions
If the user should be able to refund a payin, then the session should include the payment_report_component.create_refund
permission group:
{
"ttl": 86400,
"statements": [
{
"permissions": [
"group#payment_report_component",
+ "group#payment_report_component.create_refund"
],
"constraints": {
"merchant: {
"merchant_id": "REPLACE_ME"
}
}
}
]
}
JavaScript bundle
Include the merchant JavaScript bundle in your frontend to access the payment report component.
<script type="module" src="https://static.rainforestpay.com/sandbox.merchant.js"></script>
Payment report component
Add the Rainforest payment report component with custom configuration designed in the component studio.
<rainforest-payment-report
session-key="REPLACE_ME"
></rainforest-payment-report>
Customize the component
Head over to the Component Studio for more attributes you can provide to customize the component's appearance and behavior.
UI customization
It's possible to provide customized UI to the Payment Report through our exposed slots.
Available Slots
Name | Description |
---|---|
details-loading-indicator | Overrides the default "Loading..." UI shown while loading the relevant Details Component |
Example:
<rainforest-payment-report>
<div slot="details-loading-indicator">
(your custom UI markup)
</div>
</rainforest-payment-report>
Column configuration
The payment report will load with a set of default columns. Simply omit the columns
prop to use the following default columns.
[
{"name": "Created", "type": "builtin", "value": "created"},
{"name": "Type", "type": "builtin", "value": "type"},
{"name": "Status", "type": "builtin", "value": "status"},
{"name": "Amount", "type": "builtin", "value": "amount"},
{"name": "Customer", "type": "builtin", "value": "name"},
{"name": "Method", "type": "builtin", "value": "method"},
{"name": "Merchant Name", "type": "builtin", "value": "merchant_name"}
]
Custom configuration
Columns can be customized to fit the needs of your platform. To override the default columns for the payment report, pass in the column specifications via a JSON payload in the columns
prop.
<rainforest-payment-report
columns='[{"name":"My Custom Column Name","type":"builtin","value":"id"}]'
></rainforest-payment-report>
A column specification needs a name
, type
, and value
.
name
- The display header name for the column.type
- Set tobuiltin
ormetadata
value
- The key corresponding to a value returned from the list payments endpoint.
Valid column values
Type | Value | Description |
---|---|---|
builtin | id | The unique identifier for this payment activity. Corresponds to the id field, representing a payin_id or refund_id . |
builtin | merchant_id | The unique merchant identifier. Corresponds to the merchant_id field. |
builtin | merchant_name | The name of the merchant. Corresponds to the merchant.name field. |
builtin | created | The datetime the payment activity was created at. Corresponds to the created_at field. |
builtin | amount | The amount of this activity. Corresponds to the amount field. |
builtin | name | The billing contact name for this activity. Corresponds to the billing_contact.name field. |
builtin | billing_contact_email | The billing contact email for this activity. Corresponds to the billing_contact.email field. |
builtin | type | The type of payment activity. Set to PAYIN or REFUND. Corresponds to the payment_type field. |
builtin | status | The status of the payment activity. Corresponds to the status field. |
builtin | method | The payment method for this activity. Corresponds to the method_type field and the last 4 digits of the card or bank account. |
builtin | expected_deposit_date | The expected deposit date for this activity. Corresponds to the expected_deposit_date field.See the payments expected deposit date guide for details and limitations on this field. |
metadata | JSONPath to metadata key | The metadata for this activity. Corresponds to the metadata field. |
Metadata column
A column can be configured to display a custom metadata value passed into the config requests for varying payment activities. The value
for type metadata
should be valid JSONPath to a corresponding metadata key.
For example, a payin created with the following metadata
{
"account": {
"id": "123",
"name": "Jane Doe",
"email": "[email protected]
}
}
could have the following column specification to display the metadata account id
{
"name": "Account ID",
"type": "metadata",
"value": "$.account.id"
}
Templating columns
If you need to format the metadata for presentation to your users, you can also specify a template pattern. In order to show the Account ID as above, but formatted as "Account #123". You could specify a template string, and the pattern {{value}}
will be replaced with the value of the field:
{
"name": "Account ID",
"type": "metadata",
"value": "$.account.id",
"template": "Account #{{value}}"
}
Hyperlinks
The template can also be valid HTML in order to make a metadata column a hyperlink. For example, in order to make an email a mailto hyperlink:
{
"name": "Email",
"type": "metadata",
"value": "$.account.email",
"template": "<a style=\"color:blue;text-decoration:underline;\" href=\"mailto:{{value}}\">{{value}}</a>"
}
Data filters
The payment report can be pre-loaded with a defined set of filters, including sort by and sort order. Data filters are defined by a JSON payload of key-value pairs passed into the data-filters
prop.
A valid key
includes any of the query params from the list payments endpoint. To pass multiple values for a given key, the value can be defined as an array of values.
{
"merchant_id": "mid_123",
"status": ["PROCESSING", "FAILED"],
"sort_by": "created_at",
"sort_order": "desc"
}
Data filters will always be applied to the report and any additional filtering via the component will be in addition to the filters passed in.
Component session contraints
If the component session includes constraints to a specific resource, such as a merchant, then the data filters must be at least as restrictive. Component sessions will not filter reporting and you must define the necessary constraints via data filters. This will constrain the reporting component to only load the data that the session permissions has access to.
For example, if the payment report should only show payments for a specific merchant, then the component session should be constrained to a merchant:
{
"permissions": ["group#payment_report_component"],
"constraints": {
"merchant": {
"merchant_id": "REPLACE_ME"
}
}
}
And the payment report should pre-load the list of payments filtered to the specific merchant:
<rainforest-payment-report
session-key="REPLACE_ME"
data-filters={"merchant_id": "REPLACE_ME"}
></rainforest-payment-report>
If the report is attempting to load payments that the session permissions do not have access to, the request will error with a status code of 401 Unauthorized
and the response will include helpful information as to why this request is not valid:
{
"status": "ERROR",
"data": null,
"errors": [
{
"field": "Authorization",
"code": "AuthUnauthorized",
"message": "Permission denied: Request parameter not specified for constraint merchant"
}
]
}
Metadata
Metadata can be utilized to constrain the payment report to payments that share a common metadata value.
For example, if the payment report should only show payments for a specific account defined by the platform, then the component session can be constrained to a metadata account_id
defined on the payin:
{
"permissions": ["group#payment_report_component"],
"constraints": {
"payin": {
"metadata": {
"account_id": "123"
}
}
}
}
And the payment report should pre-load the list of payments filtered to the account_id
:
<rainforest-payment-report
session-key="REPLACE_ME"
data-filters={"metadata[$.account_id]": "123"}
></rainforest-payment-report>
Payin metadata is persisted to the related payments, such as refunds, chargebacks, and ACH returns. Constraining a session to a payin metadata field will grant permissions to view all payments (payins, refunds, chargebacks, and ACH returns) with the specified metadata.
Export payments
Payments may be exported to a comma-separated values (CSV) file. By setting the show-export-button
attribute on the payment report component, an export button will appear on the top right of the component.
<rainforest-payment-report
session-key="REPLACE_ME"
show-export-button
></rainforest-payment-report>
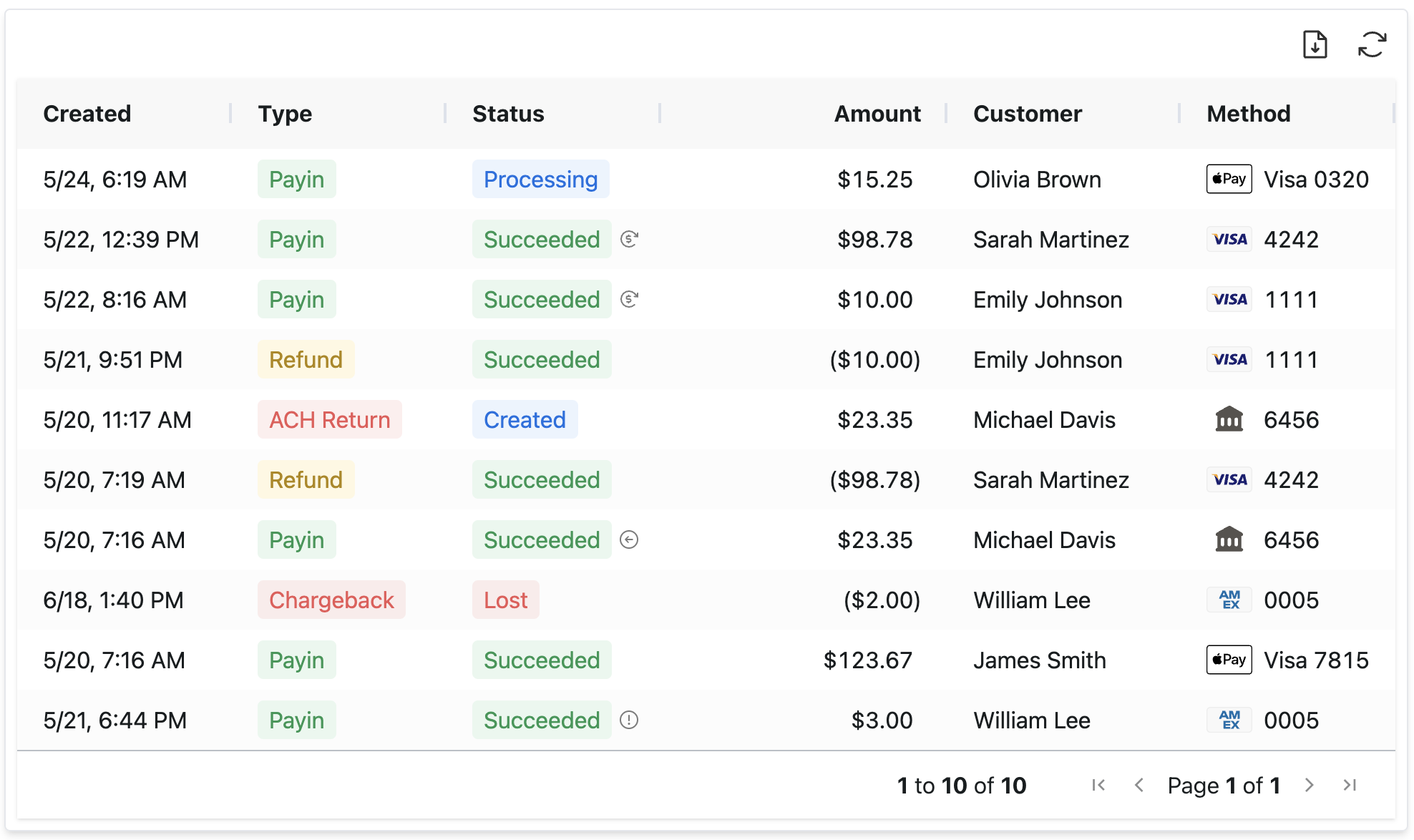
Export button displayed at the top right
Date ranges
Default date ranges for the previous or current month may be selected. If a custom date range is chosen, a maximum of 31 days may be exported.
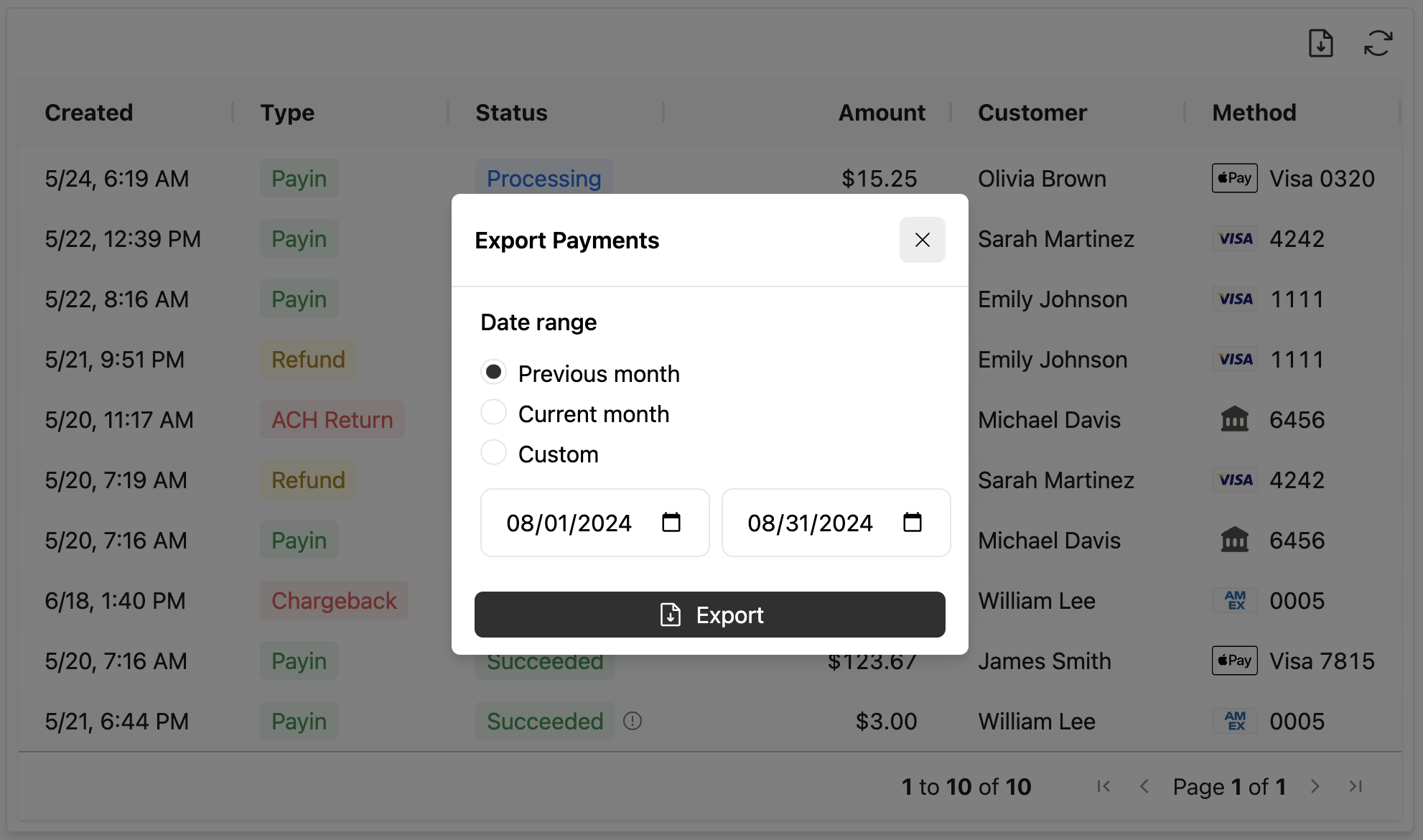
Payment report export modal
Payment filters
Any filters applied when the user is interacting with the payment report will be automatically applied to the exported payment report data. These filters can be cleared by utilizing the "Clear filters" link before clicking "Export".
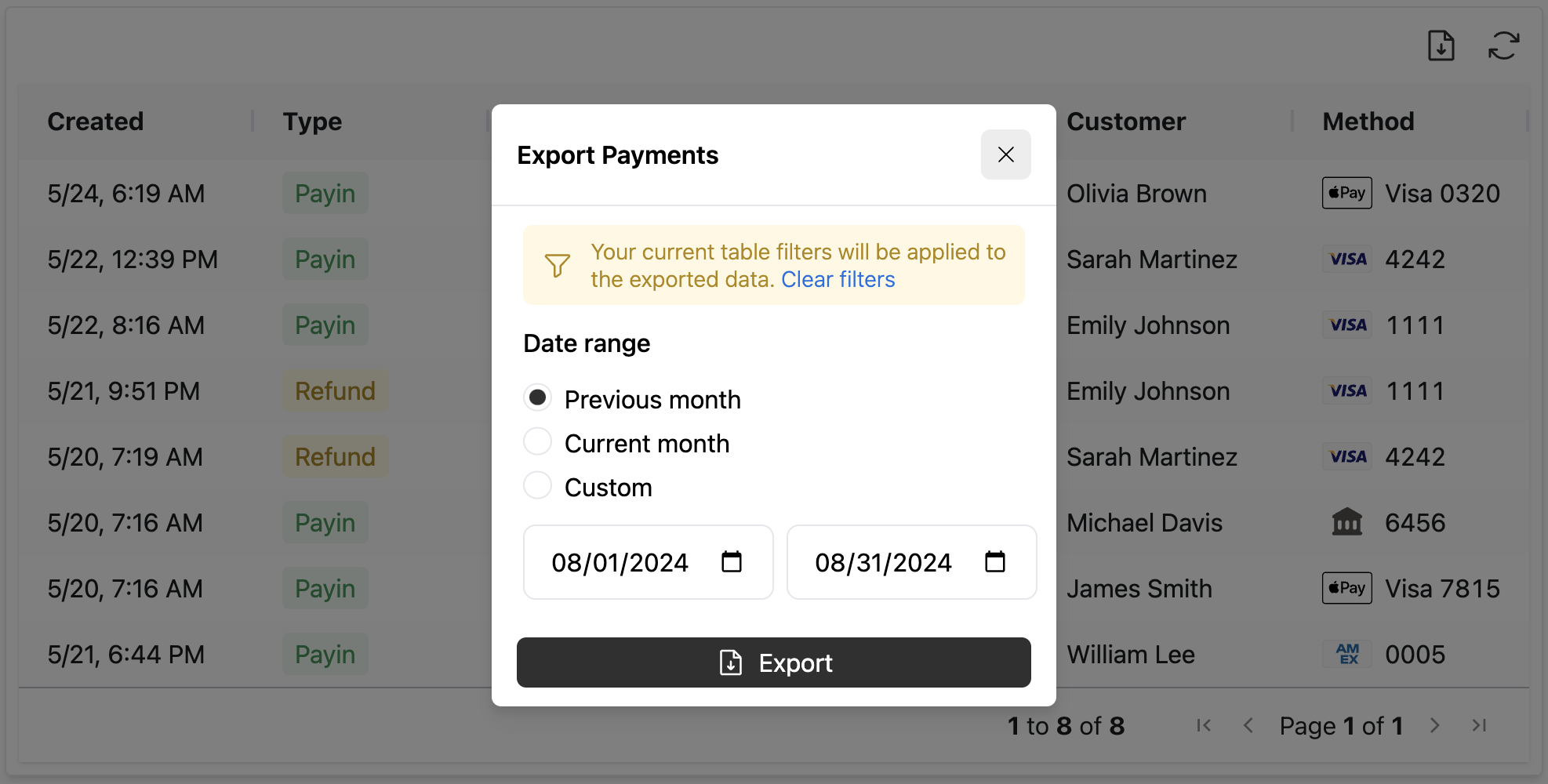
Any filters will be applied to the payment report export
Exported data
The generated CSV file will contain all the columns configured in the payment report component.
If the file will not contain any results, the modal will inform you and you may adjust your date range as needed and click "Export" again.
Updated 16 days ago