Customizing the payment component
Customizing the Payment Component for enhanced capabilities
Once you can process an online payment or store a payment method with your Payment Component integration, there are various ways to customize the payment component in your payment flow.
Hide the submit button
Integrate the Payment Component with your own submit button to have greater control over the payment flow.
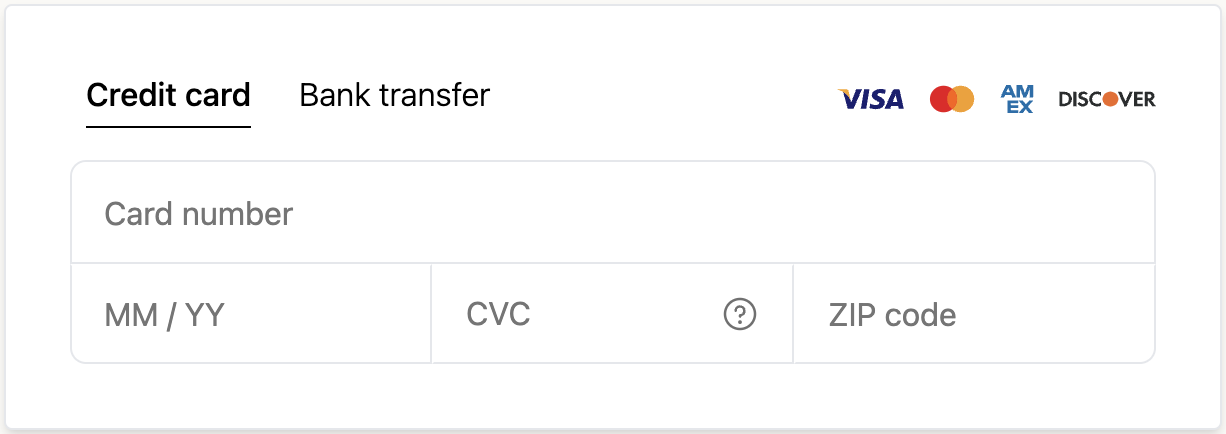
→ Head over to the hide the submit button guide on how to implement your own submit button.
Update the payment configuration
Update the payment configuration, such as the amount or billing contact details, provided to the Payment Component without having to re-render the component.
→ Head over to the update the payment configuration guide on how to update the configuration for payment processing.
Updated 3 months ago