Payin details component
Embed the Rainforest payment details component
The payin details component can be displayed to a Merchant's user or a Platform's user. The component can include payment actions, such as refunding a payin. The component can be configured to display or hide as many details as necessary.
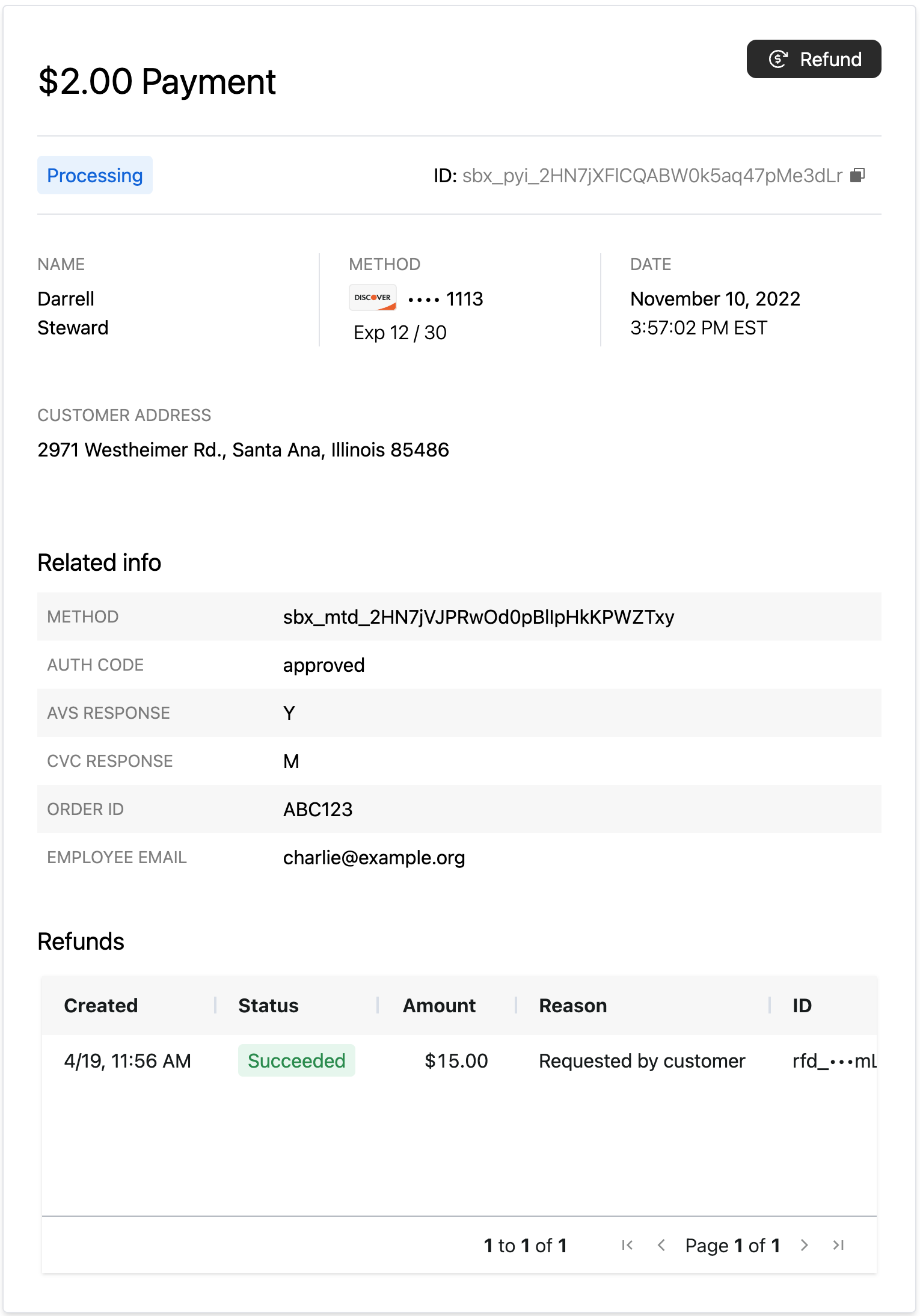
How to embed payin details
Session authentication
Create a session to grant the details component permissions to view a payin. The session should include at least the following to view the payin details:
curl --location 'https://api.rainforestpay.com/v1/sessions' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {{api_key}}' \
--data '{
"ttl": 86400,
"statements": [
{
"permissions": ["group#payin_details_component"]
}
]
}'
If the user should be able to perform actions, such as refunding a payin, then update permissions are required. The session should include at least the following:
curl --location 'https://api.rainforestpay.com/v1/sessions' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer {{api_key}}' \
--data '{
"ttl": 86400,
"statements": [
{
"permissions": [
"group#payin_details_component",
"group#payin_details_component.create_refund"
]
}
]
}'
JavaScript bundle
Include the merchant JavaScript bundle in your frontend to access the payin details component.
<script type="module" src="https://static.rainforestpay.com/sandbox.merchant.js"></script>
Payin details component
Add the Rainforest payin details component with custom configuration designed in the component studio.
A valid Payin ID must be provided in order to render the details for the payin.
<rainforest-payin-details
session-key="REPLACE_ME"
payin-id="REPLACE_ME"
></rainforest-payin-details>
Customize the component
Head over to the Component Studio for more attributes you can provide to customize the component's appearance and behavior.
Linking payment detail components
The payin details component can include data on associated payment types, such as refunds, chargebacks, or ACH returns.
When using the Payment report component, Rainforest will automatically handle opening additional payment detail components when the user is interacting with the payin details. For example, if the payin has a refund, the user can click on the refund and the refund details component will open.
If the payin details component is embedded separately from the payment report component, the interaction between the various payment detail components can be enabled by listening to event listeners.
Event listeners
The payin details component will emit the following events as the user interacts with it.
Event | Description |
---|---|
close-button-clicked | User clicked close button within one of the payment details components. The hosting page should react by hiding the corresponding payment details component or removing it from the DOM. |
view-refund | User clicked a call to action within the payin details component to display a related refund. The refund ID will be passed on the event. The hosting page should react by showing a refund details component. |
view-chargeback | User clicked a call to action within the payin details component to display a related chargeback. The chargeback ID will be passed on the event. The hosting page should react by displaying a chargeback details component. |
view-ach-return | User clicked a call to action within the payin details component to display a related ACH return. The ACH return ID will be passed on the event. The hosting page should react by displaying an ACH return details component. |
view-payment | User clicked a call to action within one of the payment details components to display a related payin. The payin ID will be passed on the event. The hosting page should react by displaying a payin details component . |
You may listen for the events above as needed. In the case of view-refund
, view-chargeback
, view-ach-return
, and view-payment
you can obtain the corresponding ID value from the event data. The identifier provided can be used to show the refund, chargeback, and ACH return detail components, respectively.
Sample event listening
var component = document.querySelector('rainforest-payin-details');
component.addEventListener('view-refund', function (event) {
// Get refund ID and display refund details component
const [refundId] = event.detail;
});
component.addEventListener('view-chargeback', function (event) {
// Get chargeback ID and display chargeback details component
const [chargebackId] = event.detail;
});
component.addEventListener('view-ach-return', function (event) {
// Get ACH return ID and display ACH return details component
const [achReturnId] = event.detail;
});
component.addEventListener('view-payment', function (event) {
// Get payin ID and display payin details component
const [payinId] = event.detail;
});
Detailed refusal reason for failed payins
During the processing of the payin, if the payin fails to process a Refusal Reason is returned in the API and displayed in the Payin Details Component. The refusal reason returns in the API in the fields refusal_code
and refusal_desc
. The refusal reason can return as a generic "Declined", which does not give context into why it failed to process.
Within 5 minutes of the payin returning as a failure, a Detailed Refusal Reason will be populated in the API and in the Payin Details Component. For example, the refusal reason could be "Declined", but the detailed refusal reason could be more descriptive, such as "Insufficient funds" or "Do not honor". For a full list of refusal reasons, see the refusal codes guide.
Payin Details Component
The DETAILED REFUSAL REASON
in the Related info section will display as "--" until it is populated.
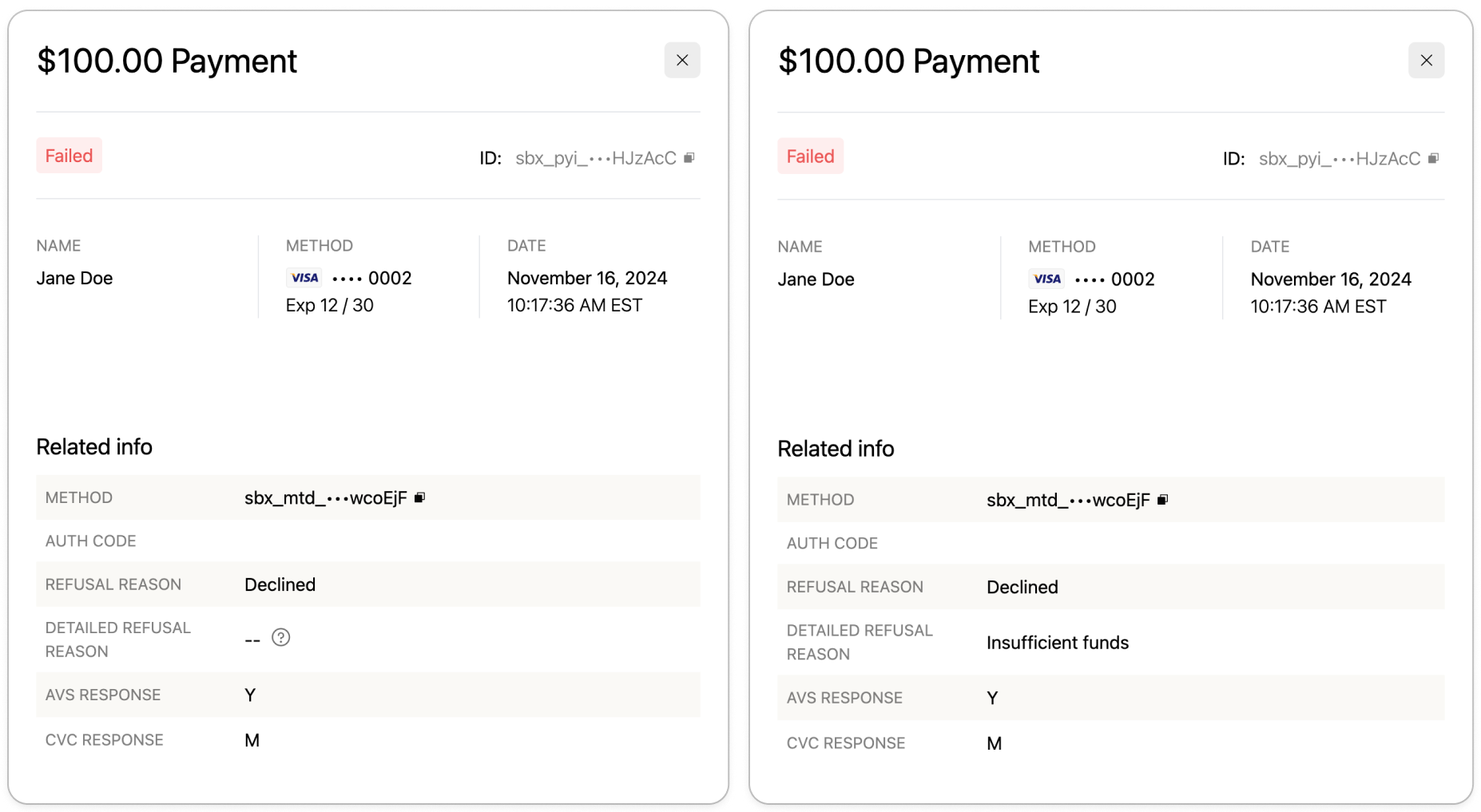
API
The following fields will be available in the list payments, list payins, and get payin endpoints to help you understand why a payin fails.
detailed_refusal_code
detailed_refusal_desc
These fields will be null
until they are populated, which can take up to 5 minutes after the payin fails.
Updated 2 months ago