Process payins via device
Process payins using a device
Once a device is registered to a merchant, it's ready to process payins.
Processing payins involves three steps:
- Create a payin config via the Rainforest API to send information about the payin (amount, user billing contact, metadata, etc.)
- Present the payin on the device
- Listen to webhooks or poll the API to know the result of the payin
Configure the payin
First, create the payin config to configure a payin with information, such as an amount and billing contact, before collecting the sensitive payin details (i.e the card number) via the device.
The merchant ID on the payin config must match the merchant the device is registered to.
With the payin config, you can also specify if you'd like to collect a signature from the User on the device, if the device supports signature collection.
The payin_config_id
from the response will be used to present the payin on the device in the next step.
{
"status": "SUCCESS",
"data": {
"payin_config_id":"cfg_2SnJJEMoszIxbP0kDYMmi5L6bai"
// ... other attributes of the payin config
}
}
Present the payin on the device
Present the payin on a registered device by providing the device registration ID and payin config ID:
// POST https://api.rainforestpay.com/v1/device_registrations/{{device_registration_id}}/payin
{
"payin_config_id": "{{payin_config_id}}"
}
Success
If the payin is successfully presented on the device, the response will include a payin_id
for the payin currently presented on the device and the status of the payin will be Presenting
.
{
"status": "SUCCESS",
"data": {
"payin_id":"pyi_2pic60IODoGBzCz8x3wN8USjvrO",
"status": "PRESENTING"
// ... other attributes of the payin
}
}
Error
There are scenarios where Rainforest is not able to present the payin on the device. If this occurs, the request will return with a status code 400 bad request. The following are some reasons the request may error:
Reason | Description |
---|---|
Device in use | The device is currently presenting another payin or payment method at this time. |
Invalid device registration | The device registration ID is either not a registered device or the merchant ID associated with the device does not match the merchant ID on the payin config. |
Device payment methods are restricted to a single merchant
Devices are registered to a single merchant and payment methods stored via a device can only process payins on the merchant associated with the device.
Cancel the payin on the device
The device interaction can be canceled in two ways:
- The user presses the cancel button the device
- The merchant requests the cancel via the Rainforest API cancel endpoint
Canceling a payin presented on a device via the Rainforest API is a best effort attempt. Rainforest cannot guarantee the presented payin can be canceled because the user may have already tapped or swiped their card on the device.
You should listen to webhooks or query the API to know the final status of the payin. If we were successfully able to cancel the payin, then the payin status will move to Canceled.
If we were unable to cancel the payin and the payin status moves to Processing, then you will need to void the payin before the daily cutoff . If you do not void the payin, then the payin will process and be deposited to the merchant.
Listen to webhooks
Because the interaction on the device is asynchronous, you will need to register webhook listeners to get the final status of the payin. You will receive a payin.processing
, payin.failed
, or payin.canceled
event once the payment flow on the device has completed:
Webhook | Payin Status | Description |
---|---|---|
payin.processing | PROCESSING | The device interaction completed successfully and issuing bank approved the payin. The user saw a successful notification on the device and the funds will move from the user's issuing bank to the merchant. |
payin.failed | FAILED | The device interaction completed, but the payin failed to process. Most likely, this means that the user tried to pay with a card, but it was declined. Details for the failure can be found in the webhook response body. You can present the same payin_config_id on the device again to retry the payment. |
payin.canceled | CANCELED | The device interaction was canceled by the user on the device or by the cancel endpoint via the API. The same payin_config_id can be used to attempt to present the payin to the device again. |
Your system should handle these events and direct your merchant accordingly:
- On a successful payin, you should show success to whoever is operating the physical device. You might also then ship an order, provision a service, or mark something paid in an accounting system.
- On a failed payin, make sure you allow the option to re-present the payment on the device in your point-of-sale or other system. Or you may need to contact the user and have them pay in some other way.
Query for the payin status
An alternative to listening to webhooks would be to query the Rainforest API get payin endpoint to check when the status of the payin updates from Presenting
to the final status.
The final status of the payin will be one of Processing, Failed, or Canceled.
Processing
Processing status means the device interaction completed successfully and issuing bank approved the payin. The user saw a successful notification on the device and the funds will move from the user's issuing bank to the merchant.
When querying the API, there is an interim status of Authorized before the payin moves to Processing. This is because, by default, Rainforest completes a single step authorization and capture payment flow.
You should continue polling the API until the payin moves to the status of Processing.
Failed
The device interaction completed, but the payin failed to process and the payin status is Failed. Most likely, this means that the user tried to pay with a card, but it was declined. Details for the failure can be found in the refusal code. You can present the same payin_config_id
on the device again to retry the payment.
Canceled
The device interaction was canceled by the user on the device or by the cancel endpoint via the API. The payin status is Canceled. The same payin_config_id
can be used to attempt to present the payin to the device again.
Payin device data
Payins processed with a device will return device data in the get payin endpoint and be displayed in the Payin Details Component in the Related info section.
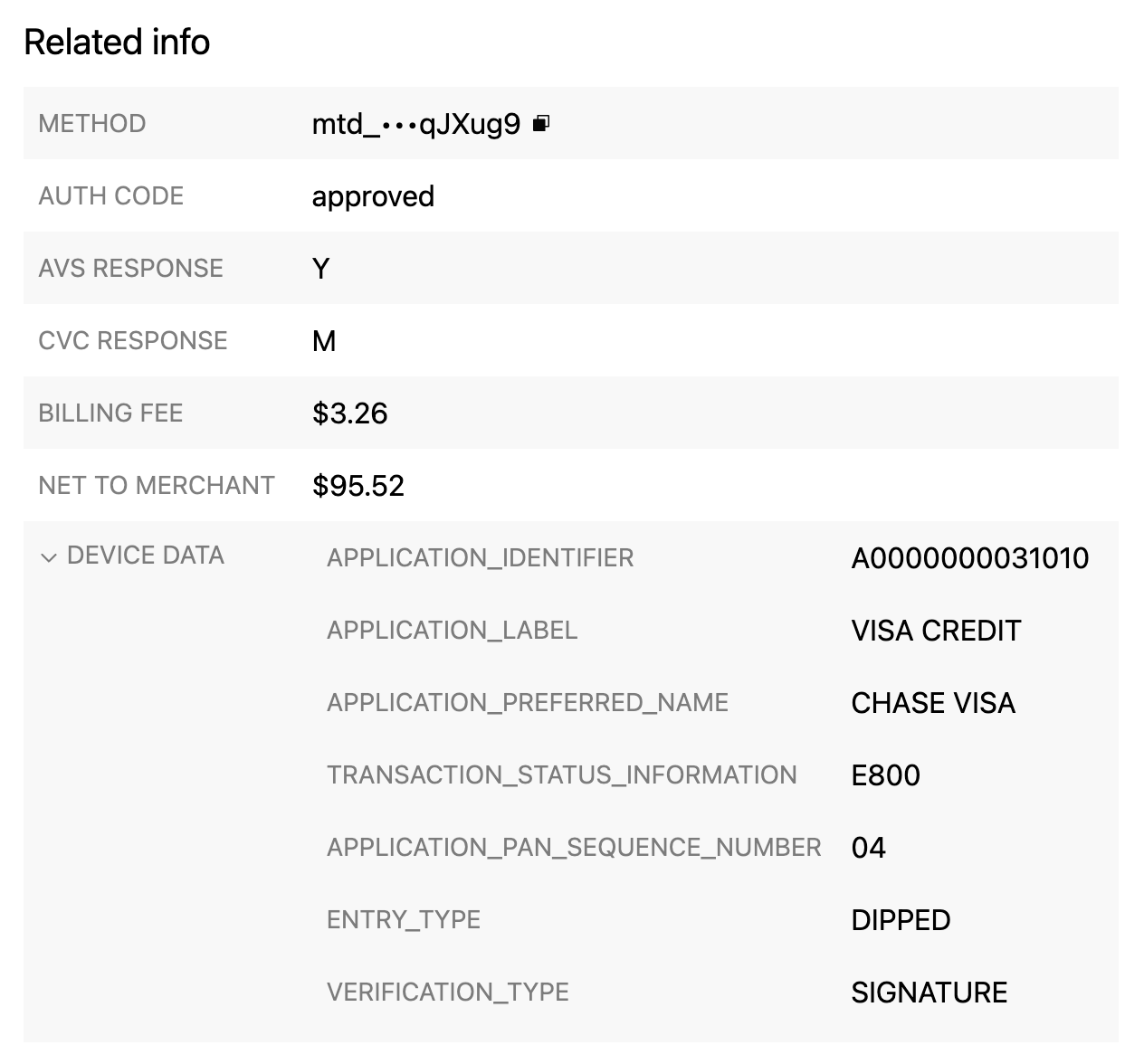
Payin Details Component - Related info section
Sandbox simulation
Rainforest does not support physical devices in the Sandbox environment, . All device payment flows can be simulated in the Rainforest Sandbox environment utilizing a virtual device.
Device interactions
Once the payin has been presented on the device, the next step is asynchronous because you are waiting for the interaction to complete on the device.
On a physical device in Production, the user would tap or swipe their card on the device to complete the payment flow, or press the cancel button to cancel the payment flow.
On the virtual device in Sandbox, you can simulate various scenarios with the simulate device interaction endpoint. For example, to test the case where a Visa card is tapped on the device and then successfully processes a payment, you would pass the event PRESENTED
and the card VISA_SUCCESS
:
{
"event": "PRESENTED",
"card": "VISA_SUCCESS"
}
Deposit and payments to succeeded
In Production, a payin will stay in the Processing status until it is available for a merchant's deposit. This can take a variable amount of time, depending on your platform's risk policies, weekends and holidays, and other factors. See the deposit timing for payments guide to understand when payments move from Processing to Succeeded and are available for deposits.
In sandbox, time does not automatically pass, enabling you to test various payment flows on demand. Call the simulate merchant deposit creation endpoint for a merchant to test their next deposit. Simulating a deposit will do the following:
- Take all of the merchant's payins, refunds, and other payments in the
Processing
status and move them to theSucceeded
status. - Process a Deposit that would be sent to the merchant.
Once the payments are in the Succeeded status, actions such as refunding can be taken on the payins.
Updated 4 months ago