Hide the submit button
Control how and when the Payment Component is submitted
When the Payment Component is shown as part of a larger form, you may want greater control, either to style your own submit button to match the rest of your site, or to control when the component is submitted.
This guide will explain how to:
- Hide the Rainforest-controlled submit button
- Know if the payment is ready to be submitted
- Submit the payment for processing from your own code
Hide the submit button
First, render the component with the hide-button
attribute, so that it no longer renders the Rainforest-controlled button. Then render your own submit button and add appropriate styling and behavior to it.
<rainforest-payment
session-key="REPLACE_ME"
payin-config-id="REPLACE_ME"
+ hide-button
></rainforest-payment>
+ <input type="submit" id="submit" disabled>
Know if the payment is ready to be submitted
In order to decide when your submit button is enabled, you'll want to wait until both your part of the form and the Payment Component are valid. The Payment Component will emit the following events while the customer is interacting with the payment fields to know if the payment is ready to be submitted or not.
Events
Event | Description |
---|---|
valid | The payment form is valid and can be submitted for processing. |
invalid | The payment form is invalid and cannot be submitted for processing. |
You should listen to the valid
and invalid
events and combine that with your own validation logic, to decide when to enable your submit button:
var component = document.querySelector('rainforest-payment')
var submit = document.querySelector('#submit')
component.addEventListener('valid', (e) => {
submit.removeAttribute('disabled');
});
component.addEventListener('invalid', (e) => {
submit.setAttribute('disabled', true);
});
Submit the payment
Once the payment is ready to be submitted and the customer has hit your submit button, you can call the Payment Component's submit
method.
component.submit();
If the payment form is valid, then Rainforest will attempt to process the payment. The Payment Component will emit the following events to let you know the status of processing.
Events
Event | Description |
---|---|
attempted | Submit button was clicked and a request will be made to process the payment. Additional event data is not included. |
approved | Payment was successfully processed. If the request was for processing a payin, the event data will include The event data will include information such as the payin_id. |
declined | Payin was declined. The event data will include information such as the payin_id . You can attempt to process the payin again with the same payin config. |
error | The request errored. The event data will include information about the error. You can attempt to process the payin again with the same payin config. |
Disable your button
You should disable your submit button when the attempted
event is emitted. We are attempting to process the payment, which can take some time. It's important to disable your flow and let the customer know the payment is processing.
Find out the status of the payment
Once the payment has completed processing, you should listen to the following events and direct the customer to the appropriate next step.
Approved
If the payment processes successfully, the approved
event will be emitted. On an approval, you should show your customer a success message and possibly a receipt, then forward them to the next step in your flow.
Declined
If the payment fails to process, the declined
event will be emitted. On a decline, the Payment Component will render a message letting the customer know what happened and prompting them to try again.
On this event, be sure to enable your submit button to allow the customer to re-attempt processing.
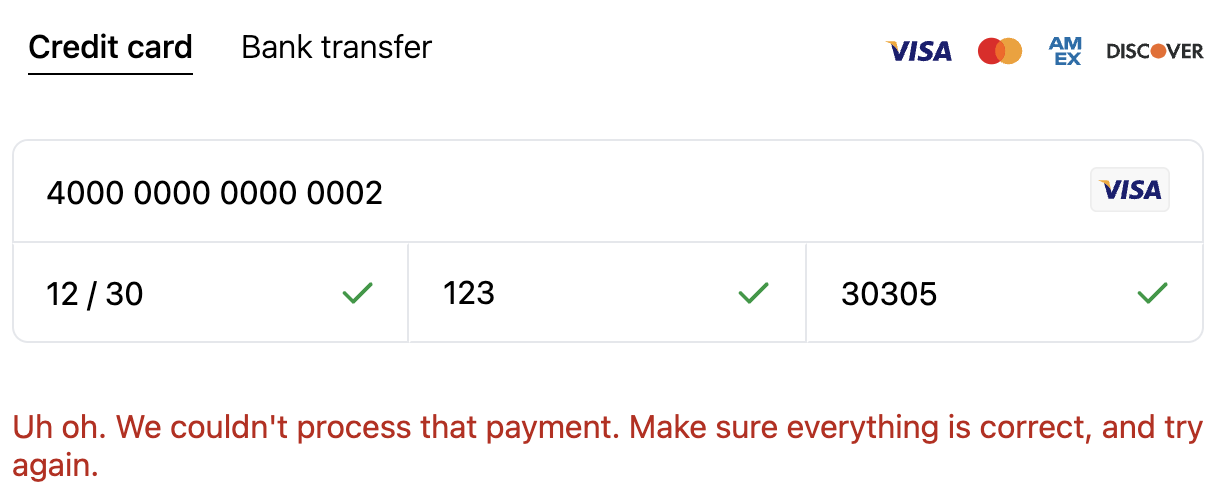
Error
If the payment errors for an unexpected reason, the error
event will be emitted. On an error, the Payment Component will render a message letting the customer know what happened and prompting them to try again.
Similar to the decline flow, be sure to enable your submit button to allow the customer to re-attempt processing.
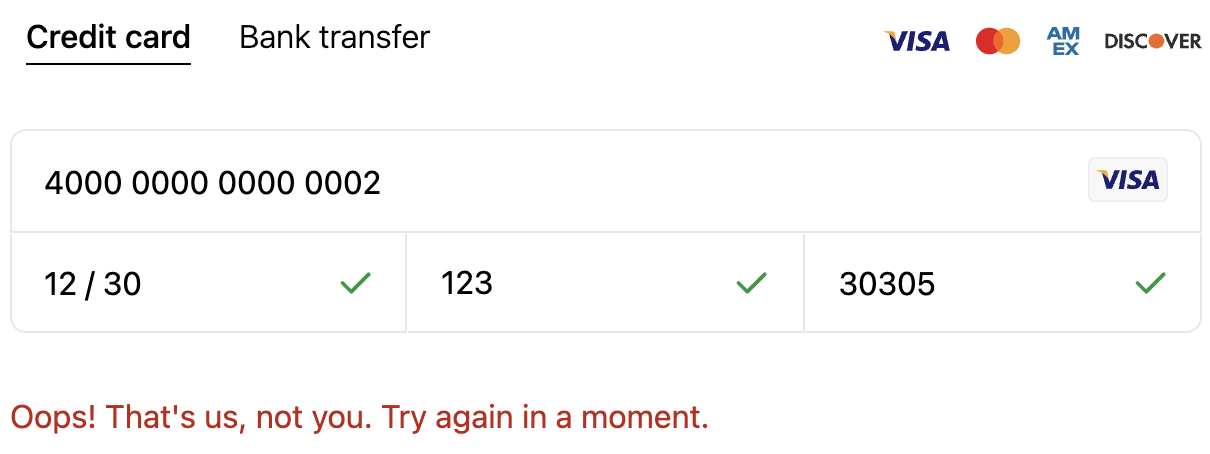
Updated about 2 months ago